Speed up the loading of your pages by using variable fonts
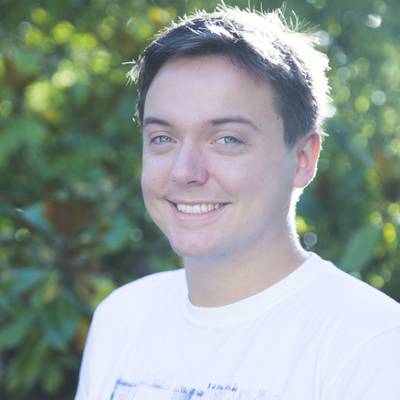
Each font weight (bold, medium, etc.) requires an additional file to be rendered properly. This means using many font variants slows down navigation... Except if you use variable fonts!
Font Weight And Style
For a given font, you can render text with different weights ("lighter", "medium", "bold", "bolder") and different styles ("italic", "oblique"). In CSS, these two aspects are governed by 2 properties:
I'll use the term font variants for different font weights, styles, or combinations of the two.
Using several font variants is super common on web pages, especially to increase the information hierarchy and guide users through large pieces of content.
Using custom font variants with web-safe fonts like Verdana or Times New Roman doesn't ask extra efforts to the browser. However, using custom font variants with a custom font (say, Raileway) poses a challenge.
Using Browser Font Variants (a.k.a. "faux bold")
Browsers can derive variants from one base font. In fact, that's what happens by default when you don't include variant fonts in the CSS.
Pros:
- Your visitors have to download only one file to display the entire text. Navigation is faster.
Cons:
- This is not standard, each browser applies its own mathematics rule to add a "faux bold" weight to each glyph of your font, or turn each glyph to make them "italic".
Browsers apply completely arbitrary rules to compute variants, and your font may become difficult to read.
In this example, the first line is "faux bold" text generated by the browser.
You can see that, for instance, the space between the letter "x" and the letter "t" in the word "text" is not optimal.
The second line uses the right bold font file and is more elegant.
Downloading Extra Files For Font Variants
In your CSS, you can define multiple files for each font variant: one for regular, one for bold, one for italic, another for bold italic... This is the most common solution.
Pros:
- Each piece of text will be easy to read and rendered as the author of the font wanted.
Cons:
- Your visitors have to download a lot of different fonts files, and this harms performance from an end-user standpoint.
Let's take an example with the famous Raleway font.
One font file weights around 60KB non-gzipped. Imagine your super beautiful website using 4 different weights: Regular, Medium, SemiBold, and Bold. Of course, some of your texts are in Italic, so you must also add: Italic Regular, Italic Medium, Italic SemiBold, and Italic Bold.
It means that you will require your visitors to download up to 8 different files, totaling 500 KB (around 350kB with gzip compression).
Both of these solutions have drawbacks. But another solution exists with only benefits. It's been available for a long time. It's called Variable fonts.
What is a Variable Font?
Variable fonts are an evolution of the OpenType font specification that enables many different variations of a typeface to be incorporated into a single file
(source: developer.mozilla.org)
Concretely, a variable font is a file that contains each glyph, just like a classic font file. It also includes a mathematical "axis" to handle weight, italic, width (and some other properties that I didn't know about and that I never had to use, like for example slant & optical size :).
Sometimes, glyphs differ between versions: e.g a letter has a different shape in italic. In this case, font authors generate another variable font file for the italic version.
Browser Support: According to caniuse.com, variable fonts are completely safe to use in all modern browsers. The support is great for 96% of the population (excluding, of course, IE11 users).
But Variable Font Files Should Be Bigger Than Traditional Font Files, Right?
Wrong! When a variable font only handles the weight axis, its file size is usually the same as a classic font file. It can be up to twice as large, but not more.
But also, Right! When a variable font handles more axes (e.g Bold + Width + Italic), the file size can be up to 5x bigger than a classic font file. But you can handle all of the possible variables with only one file!
For Raleway, my example, the author provides 2 variable fonts files (normal + italic version), which include only the weight
axis. One such font is around 120Kb (2x a classic font file)
Depending on your usage, variable fonts may not be worth it, but if you want to use multiple variations, it's definitely a good idea!
In most cases, font authors won't implement all available axes. The most used axes are weight and width.
How to Implement Variable Fonts
You can define a variable font via the @font-face
property :
@font-face {
font-family: 'MyVariableFontName';
src: url('path/to/font/file/myvariablefont.woff2') format('woff2-variations');
font-weight: 125 950;
font-stretch: 75% 125%;
font-style: normal;
}
font-weight
, in this case, is used to define boundaries. It will tell your browser that the font file is able to handle from 125 to 950.
font-stretch
will work the same but for the width
property.
font-style
in this case, my variable font doesn't handle italic style, you can also use only one value as usual.
I have also implemented the italic version:
@font-face {
font-family: 'MyVariableFontName';
src: url('path/to/font/file/myvariablefont_italic.woff2') format('woff2-variations');
font-weight: 125 950;
font-stretch: 75% 125%;
font-style: italic;
}
Implementing a Fallback
You can consider implementing a fallback for old browsers, for example, if your website has to support Internet Explorer 11 (I'm sorry for you guys !).
Most of the examples you will find on the internet explain that browsers are smart and will automatically choose the right font files. You just need to define all your variable and classic fonts, and the browsers take care of the rest.
// Variable font
@font-face {
font-family: 'MyFontName';
src: url('path/to/font/file/myvariablefont.woff2') format('woff2-variations');
font-weight: 125 950;
font-stretch: 75% 125%;
font-style: normal;
}
// Classic font definition
@font-face {
font-family: 'MyFontName';
src: url('path/to/font/file/myvariablefontregular.woff2') format('woff2');
font-weight: 400;
font-style: normal;
}
// ...And all other variations you may need to include.
// Then use it on your page
body {
font-family: 'MyFontName';
}
In my case, browsers get confused and download multiple font files instead of simply using the variable one.
To avoid this issue, I renamed my variable font family differently than my classic font, for example, Raleway VF
instead of Raleway
.
By using @supports
css rules, I can target only the browsers that support variable fonts:
// Variable font
@font-face {
font-family: 'MyFontName VF';
src: url('path/to/font/file/myvariablefont.woff2') format('woff2-variations');
font-weight: 125 950;
font-stretch: 75% 125%;
font-style: normal;
}
// Classic font definition
@font-face {
font-family: 'MyFontName';
src: url('path/to/font/file/myvariablefontregular.woff2') format('woff2');
font-weight: 400;
font-style: normal;
}
// ...And all others variation you may need to include:
// Medium, Bold, MediumItalic, BoldItalic, CondensedBoldItalic...
// Then use it on your page
body {
font-family: 'MyFontName', Arial;
}
@supports (font-variation-settings: normal) {
body {
font-family: 'MyFontName VF', Arial;
}
}
This works like a charm! You can see that my browser only downloads 260kB for my 2 variable fonts (182 KB gzipped). This is twice less than classic font files!
Overriding Variable Fonts
In some cases, you may need to apply a style only to the text displayed by a variable font. Examples include cases where the rendered text is slightly different than when you use classic fonts.
You can achieve this by using the font-variation-settings
property.
In the example below, I've just overridden the weight
property for variable font and set it to 800 instead of 600.
.semibold {
font-weight: 600;
font-variation-settings: 'wght' 800;
}
Please note that font-variation-settings
can pilot every axis you need according to its documentation.
Conclusion
Variable fonts are a very good way to reduce the size of your downloaded font files. They can help improve your page speed and reduce your website's electricity consumption.
Of course, if you want to have the most lightweight website possible, you may consider using safe web fonts, which are natively supported on every system without downloading any file. And some of these fonts are pretty cool!
And if you want to measure the impact of variable fonts on your website in terms of energy consumption and CO2 emissions, test your changes with GreenFrame.io, a tool we've built to monitor website carbon emissions. It can even run in your CI!