React-admin With Shadcn UI
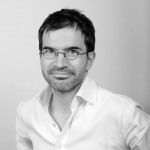
React-admin, the SPA framework for B2B apps, comes with a Material Design look and feel by default. However, react-admin is a design-agnostic framework. You can use it with any design system, including Shadcn UI.
Two Lines To Change The Look
React-admin comes with 5 built-in themes, including a theme inspired by Shadcn UI called "B&W".
To change the look of your react-admin app, you only need to change the theme
and darkTheme
props of the <Admin>
component.
import { Admin, bwLightTheme, bwDarkTheme } from 'react-admin';
export const App = () => (
<Admin
dataProvider={dataProvider}
theme={bwLightTheme}
darkTheme={bwDarkTheme}
>
{/* ... */}
</Admin>
);
You must also import the Geist font in your index.html file:
<link href="https://fonts.googleapis.com/css2?family=Geist:wght@100..900&display=swap" rel="stylesheet">
Now the application skin is Shadcn UI.
Customizing The Theme
Under the hood, react-admin still uses Material UI, so you get all the power of Material UI's theming system.
If you need to customize the theme, you can extend the bwLightTheme
and bwDarkTheme
themes. For example, to change the primary color to indigo, you can do the following:
import { Admin, bwDarkTheme } from 'react-admin';
import { deepmerge } from '@mui/utils';
// Define a custom theme by merging customizations into the base bwDarkTheme
const myTheme = deepmerge(bwDarkTheme, {
palette: {
secondary: { main: '#536dfe' },
},
});
The theme also allows you to customize components globally across the app. For example, to change the default style for all buttons:
import { Admin, bwDarkTheme } from 'react-admin';
import { deepmerge } from '@mui/utils';
// Define a custom theme with button overrides
const myTheme = deepmerge(bwDarkTheme, {
components: {
// Target the MuiButton component
MuiButton: {
defaultProps: {
// Set default variant for all buttons
variant: 'contained',
},
styleOverrides: {
// Override specific styles
root: {
// Disable text transformation (e.g., uppercase)
textTransform: 'none',
},
}
},
},
});
Finally, you can override the theme for individual component instances using the sx
prop. For example, to change the color of a specific DeleteButton:
import { DeleteButton } from 'react-admin';
const MyDeleteButton = () => (
<DeleteButton
sx={{
backgroundColor: 'red',
'&:hover': {
backgroundColor: 'darkred',
},
}}
/>
);
Refer to the react-admin theming documentation for more details on theme customization.
Headless Variant
If you prefer not to use Material UI, you can build your own set of react-admin UI components based on shadcn/ui components. This is possible thanks to the headless core of react-admin, which provides hooks and components independent of any specific UI library.
We've detailed this approach in another article, Using React-Admin With Your Favorite UI Library, demonstrating how to integrate react-admin with DaisyUI, Tailwind CSS, and React Aria.
Conclusion
React-admin is a design-agnostic framework adaptable to any design system. Whether you prefer alternatives to Material UI or want to start with a different aesthetic, react-admin allows you to build single-page applications that look and feel like Shadcn UI, DaisyUI, Mantine, Ant Design, Chakra UI, and many others.